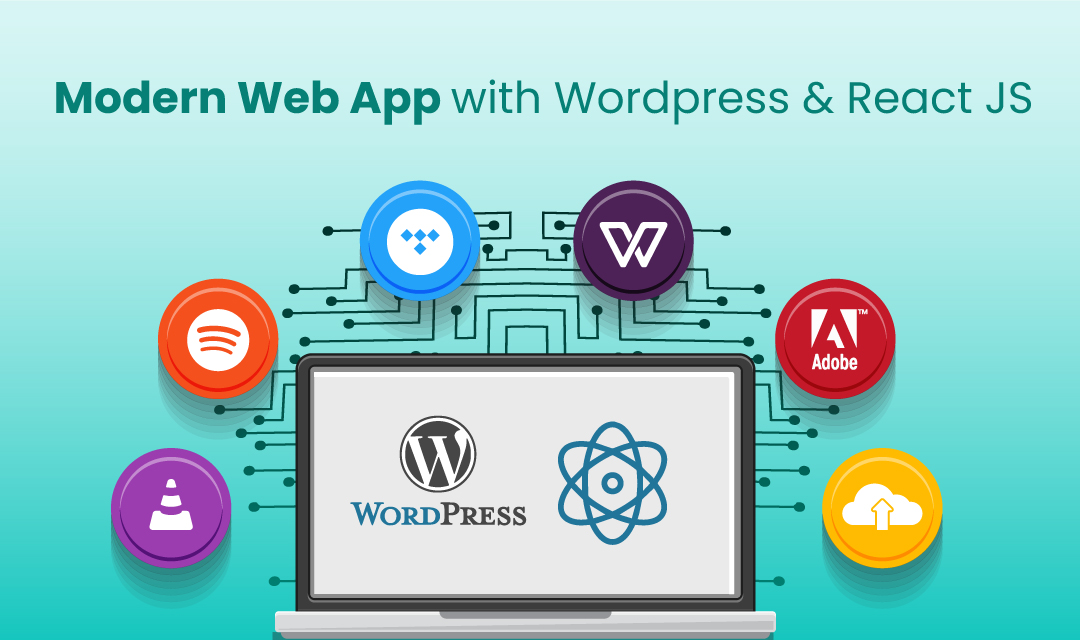
Summary: With every tech advancement, web app development dynamics are changing. WordPress & JavaScript have become popular combinations that help create the best solutions possible. Let's take a brief look at how both these inventions help in building a modern web app.
The success of Amazon, Flipkart, Alibaba, and others made us all grip the modern web app world. Online presence allows us to reach our target audience with the right services or products at the right time.
The only thing is, how will you remain reliable to your customers? For that, you need a robust architecture capable of embracing newer changes & inventions.
One such blend is WordPress and React, the JS popular. With the advent of headless CMS, this innovation has turned the heads of many experts around them. WordPress REST API is a big step taken in revolutionizing front-end development.
To use React in a WordPress site, you need to integrate WP REST API and use the popular CMS feature to unlock the potential of JavaScript.
What is WordPress REST API?
WordPress has a lot of programming jargon. So, before going any further, let's just brush our memory with these important ones & get their definitions straight.
API: The Application Program Interface is a set of protocols to build a good solution. It defines how the information is shared between programs and structures various app components to interact with each other. A good API makes program development easy by providing details in proper pieces.
REST: Representational State Transfer defines constraints on the programming ways. It provides web services which we know as RESTful APIs or REST APIs.
JSON: JavaScript Object Notation formats the data structure, making it easy for large apps to read. It makes the interaction between WordPress & any other app easy. This was made possible in WP 4.7 version. The JSON REST API has a decoupled approach that separates data (back) from views (front).
What Good Does it Do for Users?
A frontend WordPress does not have to be from 'WordPress.' One can integrate different frontend frameworks to make an intuitive user interface. It helps in engaging a targeted audience in an online venture.
WordPress has started to function better for developers by giving in features like revisions, a simple editing interface, and other familiar functionalities. One can also choose a frontend framework via REST API and display content as per his wish. Thus, he can use any potential JavaScript framework.
And so, now, one does not have to hustle around choosing an apt WP theme for an online venture. Another huge benefit is that the frontend developers can easily manage a larger project with big teams as the data is managed well with WP on the backend as one of the perks of JSON without touching PHP.
Use of React on a Headless WordPress
Such a technological development can beautify every aspect of both worlds, i.e. the user perspective & the developer's approaches to tech stacks.
WordPress REST API makes it easier to connect with other apps. A single-page app can now more easily read data on a WP by creating, editing, and deleting the same effectively.
Hire dedicated developers who do know the apt use of React in WordPress. The experts now use React to build flexible & reusable component systems, virtual DOM, effective workflow with JSX, and more.
Create great UI/UX & functionality with the best tech blend & give a modernized look to your online web app venture.
WordPress and React Demo
Herein is a small example of how to tie React with WordPress.
1. WP data querying
Assuming that you already have WP installed in your system, let's move to the actual part. Herein, there is a use of Docker Compose for local development. You can use WP REST API from your front end to make a GET request to /wp-json/wp/v2/{post type}. For instance, you can easily get all the details from /wp-json/wp/v2/posts.
Now, from the developer's frontend, the request will be made with the fetch API.
fetch('/wp-json/wp/v2/posts') .then(response => response.json()) .then(posts => console.log(posts));
2. WP REST API Querying from React
For querying such data, add the following components:
import React, { useEffect, useState } from 'react'; import Card from '@material-ui/core/Card'; import CardContent from '@material-ui/core/CardContent'; import Typography from '@material-ui/core/Typography'; import Grid from '@material-ui/core/Grid'; export default function Posts() { const [posts, setPosts] = useState([]); useEffect(() => { async function loadPosts() { const response = await fetch('/wp-json/wp/v2/posts'); if(!response.ok) { // oups! something went wrong return; } const posts = await response.json(); setPosts(posts); } loadPosts(); }, []) return ( <Grid container spacing={2}> {posts.map((post, index) => ( <Grid item xs={4} key={index}> <Card> <CardContent> <Typography color="textSecondary" gutterBottom dangerouslySetInnerHTML={{__html: post.title.rendered}} /> <Typography variant="body2" component="p" dangerouslySetInnerHTML={{__html: post.content.rendered}} /> </CardContent> </Card> </Grid> ))} </Grid> ); }
You'll see that herein; there is a use of the React Hooks useEffect and useState.
The array of posts is first declared using useState, provides a callback for updating it.
After the component is mounted, useEffect then permits running the fetch code.
Finally, you may "map" over the posts array and return components for each one of them to create a list of posts. There are a couple of peculiarities with the way useEffect functions, though:
- An async function cannot be sent straight to useEffect as the callback is unable to return a promise.
- To just execute once, useEffect needs to receive an empty array. React is informed by this that the effect is independent of all values.
How to create custom React Hook?
Create a custom React Hook with "useFetch."
import { useEffect, useState } from 'react'; export default function useFetch(url) { const [data, setData] = useState(null); useEffect(() => { async function loadData() { const response = await fetch(url); if(!response.ok) { // oups! something went wrong return; } const posts = await response.json(); setData(posts); } loadData(); }, [url]); return data; }
With the help of useEffect, you can run a fetched URL changes again. Thus, this is how one can create a custom React Hook.
export default function Posts() { const posts = useFetch('http://localhost/wp-json/wp/v2/posts'); return ( <Grid container spacing={2}> {posts && posts.map((post, index) => ( <Grid item xs={4} key={index}> <!-- this code is unchanged --> </Grid> ))} ); }
3. Adding new data type
There are many WP functions to register what’s needed. All the data will go in a new PHP file in wp-content/plugins/my_plugin.php.
Let’s start with the meta comments:-
<?php /** * @package Sample_Plugin * @version 1.0.0 */ /* Plugin Name: Sample Plugin Plugin URI: http://example.com/ Description: The beginning of an awesome plugin Author: Me Version: 1.0.0 Author URI: http://example.com/ */
Create a course with available WP options and create a custom post type. For more insights on this, you can dive into WP documentation or use a generator. Take over the necessary plugins to activate WP admin and add necessary courses.
Thus, get the list of courses in a React component with the use of useFetch Hook.
export default function Courses() { const courses = useFetch('http://localhost/wp-json/wp/v2/courses'); return ( <List component="nav" aria-label="main mailbox folders"> {courses && courses.map((course, index) => ( <ListItem key={index}></ListItem> ))} </List> ); }
4. Further customization
There are many more complex use cases than the one displayed here, but it will help to understand WP & React built web apps.
To provide some variety, I made these courses available for purchase to logged-in customers. Thus, the API must expose a price field. There are two ways to do this:
Use post meta: The cleaner approach would be to approach it from the perspective of a plugin, but implementing the meta box for modifying these fields involves a little more work.
Use custom fields: These can be inserted straight from the editor, but their exposure requires modification of the REST API.
function generate_course_type() { // ... previous code from generate_course_type $meta_args = array( 'type' => 'number', 'description' => 'The price of a course.', 'single' => true, 'show_in_rest' => true, ); register_post_meta( 'course_type', 'price', $meta_args ); }
The REST API will be automatically exposed if show _in _rest is set to true.
As an alternative, register _rest _field may be used to expose custom fields built in the WP admin interface to the REST API:
function get_price_field($object, $field_name, $value) { return floatval(get_post_meta($object\['id'])[$field_name\][0]); } function register_course_price_in_api() { register_rest_field('course_type', 'price', array( 'get_callback' => 'get_price_field', 'update_callback' => null, 'schema' => null, )); } add_action( 'rest_api_init', 'register_course_price_in_api' );
getcallback for the price field and in that callback, retrieves and formats the field from post meta.
After this, Querying the REST API returns a payload.
[ { "id": 72, "date": "2020-03-11T06:31:52", "slug": "markething-madness-with-frank", "status": "publish", "type": "course_type", "title": { "rendered": "Markething Madness with Frank" }, "content": { "rendered": "", "protected": false }, "price": 75.99, "_links": { // ... } // ... }, // ... ]
On a concluding note!
WordPress is evolving with the REST API. So, now there are even more possibilities for conquering the web app space with the most powerful JS library, React. Implement these two tech advances in your solution and get your users the best online result and experience.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment